R Programming (NEP & CBCS) Questions with Answers
5 & 10 Marks (IMP)
1. Explain different data structures.
A data structure is a particular way of organizing data in a computer so that it can be used effectively. The idea is to reduce the space and time complexities of different tasks.
1. Vectors:
- Definition: A vector is a sequence of data elements of the same basic type (numeric, character, logical, etc.).
- Usage: Vectors are commonly used for storing single-dimensional data.
- Example:
my_vector <- c(1, 2, 3, 4, 5)
2. Matrices:
- Definition: A matrix is a collection of data elements arranged in rows and columns.
- Usage: Matrices are useful for storing data in two dimensions.
- Example:
my_matrix <- matrix(1:9, nrow=3, ncol=3)
3. Lists:
- Definition: A list is a collection of data elements of different types.
- Usage: Lists can store elements of various types and sizes.
- Example:
my_list <- list(name="Alice", age=30, has_children=TRUE)
4. Data Frames:
- Definition: A data frame is a table-like structure made up of rows and columns.
- Usage: Data frames are typically used to store structured data where each column can be a different type.
- Example:
my_df <- data.frame(name=c("Alice", "Bob"), age=c(30, 25))
5. Factors:
- Definition: Factors represent categorical data where the levels (categories) are predefined.
- Usage: Factors are useful when dealing with data that has a fixed number of categories.
- Example:
my_factor <- factor(c("Low", "Medium", "High"))
6. Arrays:
- Definition: Arrays are multi-dimensional extensions of vectors in R.
- Usage: Arrays can store data in more than two dimensions.
- Example:
my_array <- array(1:12, dim=c(2, 3, 2))
7. Factors:
- Definition: Factors represent categorical data where the levels (categories) are predefined.
- Usage: Factors are useful when dealing with data that has a fixed number of categories.
- Example:
my_factor <- factor(c("Low", "Medium", "High"))
2. Explain different matrix operation function in R?
R, matrix operations refer to various functions that allow you to manipulate matrices. These operations are very useful when working with data sets organized as matrices.
cbind() and rbind()
- The
cbind()
function is used to combine matrices by binding them column-wise. - The
rbind()
function is used to combine matrices by binding them row-wise.
- The
rowSums() and colSums()
- The
rowSums()
function computes the sum of elements across rows in a matrix. - The
colSums()
function computes the sum of elements across columns in a matrix.
- The
abs()
- The
abs()
function is used to find the absolute values of the elements in a matrix.
- The
all()
- The
all()
function checks if all elements in a matrix are true.
- The
Multiplying Matrices
- To perform matrix multiplication, you can use the
%*%
operator.
- To perform matrix multiplication, you can use the
Transposing Matrices
- The
t()
function can be used to transpose a matrix.
- The
R, there are several matrix operation functions that are commonly used to perform various operations on matrices. Here are some important matrix operation functions in R:
1. Matrix Multiplication:
- Function:
%*%
- Usage: This operator is used to perform matrix multiplication between two matrices.
- Example:
A <- matrix(1:4, nrow=2) # Create a 2x2 matrix
B <- matrix(5:8, nrow=2) # Create another 2x2 matrix
result <- A %*% B # Matrix multiplication of A and B
2. Transpose:
- Function:
t()
- Usage: Transposes the rows and columns of a matrix.
- Example:
A <- matrix(1:4, nrow=2) # Create a 2x2 matrix
result <- t(A) # Transpose the matrix A
3. Determinant:
- Function:
det()
- Usage: Calculates the determinant of a square matrix.
- Example:
A <- matrix(c(1, 2, 3, -1), nrow=2) # Create a 2x2 matrix
det_A <- det(A) # Calculate the determinant of matrix A
4. Inverse:
- Function:
solve()
- Usage: Computes the inverse of a non-singular square matrix.
- Example:
A <- matrix(c(1, 2, 3, 4), nrow=2) # Create a 2x2 matrix
A_inv <- solve(A) # Calculate the inverse of matrix A
5. Eigenvalues and Eigenvectors:
- Function:
eigen()
- Usage: Computes the eigenvalues and eigenvectors of a square matrix.
- Example:
A <- matrix(c(1, 2, 3, 4), nrow=2) # Create a 2x2 matrix
eigen_A <- eigen(A) # Compute the eigenvalues and eigenvectors of matrix A
6. Diagonal Matrix:
- Function:
diag()
- Usage: Creates a diagonal matrix from a vector of diagonal elements.
- Example:
diag_matrix <- diag(c(1, 2, 3)) # Create a diagonal matrix with diagonal elements 1, 2, and 3
3. Write about plot function.
The plot() function
In R, the base graphics function to create a plot is the plot()
function. It has many options and arguments to control many things, such as the plot type, labels, titles and colors.
Syntax
The syntax for the plot() function is:
plot(x,y,type,main,xlab,ylab,pch,col,las,bty,bg,cex,…)
Parameter
Parameter | Description |
x | The coordinates of points in the plot |
y | The y coordinates of points in the plot |
type | The type of plot to be drawn |
main | An overall title for the plot |
xlab | The label for the x axis |
ylab | The label for the y axis |
pch | The shape of points |
col | The foreground color of symbols as well as lines |
las | The axes label style |
bty | The type of box round the plot area |
bg | The background color of symbols (only 21 through 25) |
cex | The amount of scaling plotting text and symbols |
… | Other graphical parameters |
Different Plot Types
You can change the type of plot that gets drawn by using the type argument. Here’s a list of all the different types that you can use.
Value | Description |
“p” | Points |
“l” | Lines |
“b” | Both points and lines |
“c” | The lines part alone of “b” |
“o” | Both points and lines “overplotted” |
“h” | Histogram like (or high‐density) vertical lines |
“s” | Step plot (horizontal first) |
“S” | Step plot (vertical first) |
“n” | No plotting |
R plot() Function:
A plot() function is a generic function that is used to plot points in a graph.
Plot One Point in R
Generally the ordered pair (x, y) represents a point on a graph.
In R, we pass specific points for x-axis and y-axis respectively as a parameter for the plot()
function to create a plot. For example,
#create one point at (2,4)
plot(2, 4)
Output
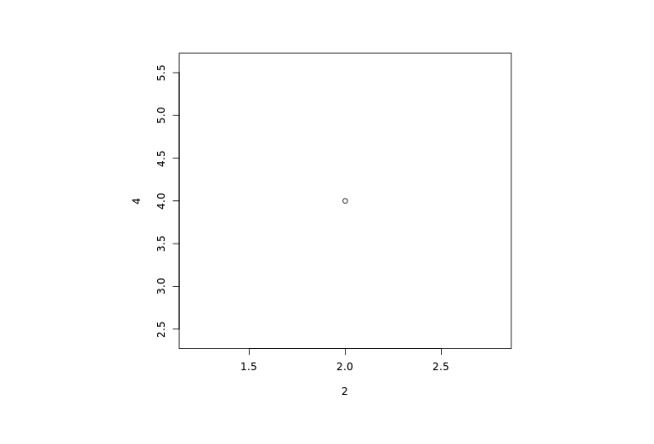
In the above example, we have used the plot()
function to plot one point on a graph.
plot(2, 4)
Here,
- 2 – specifies point on the x-axis
- 4 – specifies point on the y-axis
Plot Multiple Points in R
We can also plot multiple points on a graph in R. For that we use the R Vectors. For example,
# create a vector x
x <- c(2, 4, 6, 8)
# create a vector y
y <- c(1, 3, 5, 7)
# plot multiple points
plot(x, y)
Output
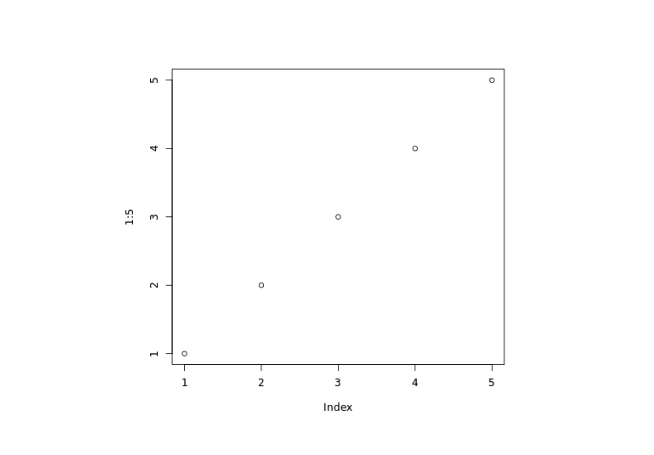
In the above example, we can plot multiple points on a graph using the plot()
function and R vector.
plot(x, y)
Here, we have passed two vectors: x and y inside plot()
to plot multiple points.
The first item of x and y i.e. 2 and 1 respectively plots 1st point on graph and second item of x and y plots 2nd point on graph and so on.
Note: Make sure the number of points on both vectors are the same.
Plot Sequence of Points in R
In R, we use the plot()
function and the: operator to draw a sequence of points. For example,
# draw sequence of points
plot(1:5)
Output
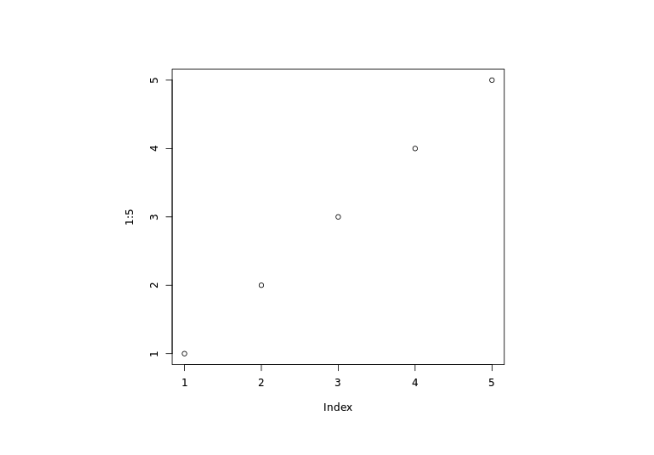
In the above example, we have used the plot()
and the :
operator to draw a sequence of points.
The plots are drawn in (1, 1), (2, 2), (3, 3), (4, 4), (5, 5) order.
Draw a Line in R
We pass the type
parameter inside the plot()
function to change the plot type. For example,
# draw a line
plot(1:5, type="l")
Output
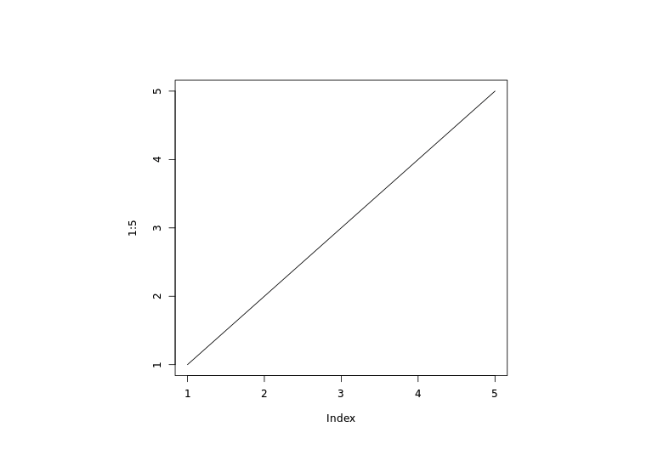
In the above example, we have used the type
parameter inside plot()
to change the type of plot.
plot(1:5, type = "l")
Here, type = "l"
draws a line to connect all the points.
4. Explain about Variables, constants and Data Types in R Programming.
In R programming, variables, constants, and data types play significant roles in storing and manipulating data efficiently. Let’s delve into each of these concepts:
Variables:
- Definition: Variables in R are used to store and manipulate data values. They act as placeholders for data that can be modified during the program execution.
- Declaration: In R, variables are typically declared by assigning a value to a name using the assignment operator
<-
or=
. - Example:
# Variable declaration
x <- 10 # Assigning the value 10 to the variable x
name <- "Alice" # Assigning a string value to the variable name
Constants:
- Definition: In R, constants are similar to variables, but their values remain fixed and do not change during program execution.
- Convention: While R does not have built-in keywords for constants, developers often use uppercase naming conventions to denote constant values.
- Example:
PI <- 3.14 # Example of a constant representing the value of Pi
Data Types:
In R, there are various data types that define the type of data that can be stored in variables. Some of the common data types include:
Numeric:
- Represents numerical data (integers or decimals).
- Example:
age <- 30
Character:
- Represents text or string data.
- Example:
name <- "Alice"
Logical:
- Represents Boolean values TRUE or FALSE.
- Example:
is_valid <- TRUE
Integer:
- Represents integer values without decimal points.
- Example:
count <- 5L
Complex:
- Represents complex numbers with real and imaginary parts.
- Example:
z <- 3 + 2i
Factor:
- Represents categorical data with fixed levels.
- Example:
grade <- factor(c("A", "B", "C"))
Date and Time:
- Represents date and time values.
- Example:
dob <- as.Date("1990-05-15")
5. How to create, name ,access , merging and manipulate list elements? Explain with examples.
To create, name, access, merge, and manipulate list elements in R, you can follow these steps. The list data structure allows you to store different types of data elements and is versatile for handling complex data structures. Here’s an explanation with examples for each operation:
1. Create a List:
You can create a list using the list()
function in R, where you can include various data elements within the list.
# Create a list
my_list <- list(name = "Alice", age = 30, has_children = TRUE)
2. Name List Elements:
When creating a list, you can assign names to each element within the list using the names()
function in R.
# Name list elements
names(my_list) <- c("person_name", "person_age", "has_kids")
3. Access List Elements:
You can access individual elements within a list using double square brackets [[ ]]
by specifying the position or the name of the element.
# Access list elements
print(my_list[["person_name"]]) # Accessing element by name
print(my_list[[2]]) # Accessing element by position
4. Merging Lists:
To merge two or more lists into a single list, you can use the c()
function.
# Merge lists
list1 <- list(a=1, b=2)
list2 <- list(c=3, d=4)
merged_list <- c(list1, list2)
5. Manipulate List Elements:
You can manipulate list elements by modifying, adding, or removing elements within the list.
# Modify list element
my_list[["person_age"]] <- 31 # Update age
# Add a new element
my_list[["city"]] <- "New York" # Add a new element
# Remove an element
my_list[["has_kids"]] <- NULL # Remove an element
6. Implement binary search tree with R.
To implement a binary search tree in R, we can define a node structure and create functions to insert, search, and traverse the tree. Here’s how you can implement a simple binary search tree in R:
# Define the structure for a node in the binary search tree
node <- function(value) {
list(
value = value,
left = NULL,
right = NULL
)
}
# Function to insert a value into the binary search tree
insert <- function(root, value) {
if (is.null(root)) {
root <<- node(value)
} else if (value <= root$value) {
if (is.null(root$left)) {
root$left <<- node(value)
} else {
insert(root$left, value)
}
} else {
if (is.null(root$right)) {
root$right <<- node(value)
} else {
insert(root$right, value)
}
}
}
# Function to search for a value in the binary search tree
search <- function(root, value) {
if (is.null(root)) {
return(FALSE)
} else if (value == root$value) {
return(TRUE)
} else if (value < root$value) {
return(search(root$left, value))
} else {
return(search(root$right, value))
}
}
# Function to perform an in-order traversal of the binary search tree
inorder <- function(root) {
if (!is.null(root)) {
inorder(root$left)
cat(root$value, " ")
inorder(root$right)
}
}
# Create a sample binary search tree
tree <- NULL
insert(tree, 5)
insert(tree, 3)
insert(tree, 7)
insert(tree, 2)
insert(tree, 4)
insert(tree, 6)
insert(tree, 8)
# Perform in-order traversal of the binary search tree
inorder(tree)
7. Explain different types of operators in R.
Operators in R are symbols that are used to perform various operations on variables, values, and data structures. Here are the main types of operators in R:
- Arithmetic Operators:
- Addition (+): Adds two values.
- Subtraction (-): Subtracts the right operand from the left operand.
- Multiplication (*): Multiplies two values.
- Division (/): Divides the left operand by the right operand.
- Exponentiation (^): Raises the left operand to the power of the right operand.
- Modulo (%%): Returns the remainder of the division of the left operand by the right operand.
- Relational Operators:
- Equal to (==): Checks if two values are equal.
- Not equal to (!=): Checks if two values are not equal.
- Greater than (>): Checks if the left operand is greater than the right operand.
- Less than (<): Checks if the left operand is less than the right operand.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right operand.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right operand.
- Logical Operators:
- AND (&&): Returns TRUE if both operands are TRUE.
- OR (||): Returns TRUE if at least one operand is TRUE.
- NOT (!): Negates the logical value of the operand.
- Assignment Operators:
- Assignment (=): Assigns a value to a variable.
- Compound Assignment (+=, -=, *=, /=): Assigns the result of an operation to a variable.
- Special Operators:
- Colon Operator (:): Creates a sequence of values.
- %in%: Checks if a value is present in a vector or list.
- %*%: Matrix multiplication operator.
- Arithmetic Operators:
Arithmetic Operators:
Arithmetic operations in R simulate various math operations, like addition, subtraction, multiplication, division, and modulo using the specified operator between operands, which may be either scalar values, complex numbers, or vectors. The R operators are performed element-wise at the corresponding positions of the vectors.
Logical Operators:
Logical operations in R simulate element-wise decision operations, based on the specified operator between the operands, which are then evaluated to either a True or False boolean value. Any non-zero integer value is considered as a TRUE value, be it a complex or real number.
Relational Operators:
The relational operators in R carry out comparison operations between the corresponding elements of the operands. Returns a boolean TRUE value if the first operand satisfies the relation compared to the second. A TRUE value is always considered to be greater than the FALSE.
Assignment Operators:
Assignment operators in R are used to assigning values to various data objects in R. The objects may be integers, vectors, or functions. These values are then stored by the assigned variable names. There are two kinds of assignment operators: Left and Right.
Miscellaneous Operators:
These are the mixed operators in R that simulate the printing of sequences and assignment of vectors, either left or right-handed. (%in% Operator)
8. Explain control statement in R.
Control Statements are expressions used to control the execution and flow of the program based on the conditions provided in the statements. These structures are used to make a decision after assessing the variable.
In R-Programming, there are 8 types of control statements as follows:
- if condition.
- if-else condition
- for loop
- nested loops
- while loop
- repeat and break statement
- next statement
This control structure checks the expression provided in parenthesis is true or not. If true, the
execution of the statements in braces {} continues.
Syntax:
if(expression)
{
statements
....
....
}
Example:
x <- 100
if(x > 10)
{
print(paste(x, "is greater than 10"))
}
Output:
[1] "100 is greater than 10"
It is similar to if condition but when the test expression in if condition fails, then statements in else condition are executed.
Syntax:
if(expression)
{
statements
....
}
else
{
statements
....
}
Example:
x <- 5
# Check value is less than or greater than 10
if(x > 10)
{
print(paste(x, "is greater than 10"))
}
else
{
print(paste(x, "is less than 10"))
}
Output:
[1] "5 is less than 10"
It is a type of loop or sequence of statements executed repeatedly until exit condition is reached.
Syntax:
for(value in vector)
{
statements
....
}
Example:
x <- letters[4:10]
for(i in x)
{
print(i)
}
Output:
[1] "d"
[1] "e"
[1] "f"
[1] "g"
[1] "h"
[1] "i"
[1] "j"
Nested loops are similar to simple loops. Nested means loops inside loop. Moreover, nested loops are used to manipulate the matrix.
Example:
# Defining matrix
m <- matrix(2:15, 2)
for (r in seq(nrow(m)))
{
for (c in seq(ncol(m)))
{
print(m[r, c])
}
}
Output:
[1] 2
[1] 4
[1] 6
[1] 8
[1] 10
[1] 12
[1] 14
[1] 3
[1] 5
[1] 7
[1] 9
[1] 11
[1] 13
[1] 15
while loop is another kind of loop iterated until a condition is satisfied. The testing expression is checked first before executing the body of loop.
Syntax:
while(expression)
{
statement
}
Example:
x = 1
# Print 1 to 5
while(x <= 5)
{
print(x)
x = x + 1
}
Output:
[1] 1
[1] 2
[1] 3
[1] 4
[1] 5
repeat is a loop which can be iterated many number of times but there is no exit condition to
come out from the loop. So, break statement is used to exit from the loop. break statement can
be used in any type of loop to exit from the loop.
Syntax:
repeat
{
statements
if(expression)
{
break
}
}
Example:
x = 1
# Print 1 to 5
repeat
{
print(x)
x = x + 1
if(x > 5)
{
break
}
}
Output:
[1] 1
[1] 2
[1] 3
[1] 4
[1] 5
return statement is used to return the result of an executed function and returns control to the calling function.
Syntax:
return(expression)
Example:
# Checks value is either positive, negative or zero
func<- function(x)
{
if(x > 0)
{
return("Positive")
}
else if(x < 0)
{
return("Negative")
}
else
{
return("Zero")
}
}
func(1)
func(0)
func(-1)
Output:
[1] "Positive"
[1] "Zero"
[1] "Negative"
next statement is used to skip the current iteration without executing the further statements and continues the next iteration cycle without terminating the loop.
Example:
# R program to illustrate the use of next statement
#create vector
x<- 1:10
# using for loopto iterate over the sequence
for (i in x)
{
# checking condition
if (i == 3)
{
# using next keyword
next
}
# displaying items in the sequence
print(i)
}
Output:
[1] 1
[1] 2
[1] 4
[1] 5
9. Write a R program to implement quicksort.
# Define the quicksort function
quicksort <- function(arr)
{
if (length(arr) <= 1)
{
return(arr)
}
pivot <- arr[1]
smaller <- arr[arr < pivot]
equal <- arr[arr == pivot]
larger <- arr[arr > pivot]
return(c(quicksort(smaller), equal, quicksort(larger)))
}
# Example usage
input_array <- c(7, 2, 1, 6, 8, 5, 3, 4)
sorted_array <- quicksort(input_array)
cat("Sorted array:", sorted_array, "\n")
10. Write about user defined functions in R with suitable example?
Example of a User-Defined Function in R:
Let’s create a simple user-defined function in R to calculate the factorial of a given number.
# Define the user-defined function to calculate factorial
calculate_factorial <- function(n) {
if (n == 0 | n == 1) {
return(1)
} else {
return(n * calculate_factorial(n - 1))
}
}
# Call the user-defined function to calculate factorial
number <- 5
factorial_result <- calculate_factorial(number)
cat(paste("The factorial of", number, "is", factorial_result))
In this example:
- We define a user-defined function called
calculate_factorial
that takes one argumentn
representing the number for which the factorial needs to be calculated. - Inside the function, we use a conditional statement to handle the base case (factorial of 0 or 1) and the recursive case where the factorial is calculated using the formula
n * calculate_factorial(n - 1)
. - Then, we call the
calculate_factorial
function with the number5
and store the result infactorial_result
. - Finally, we print the calculated factorial using the
cat
function.
Benefits of User-Defined Functions:
- Code Reusability: User-defined functions allow you to encapsulate logic that can be reused across your script or program. This promotes code reusability and makes the code easier to maintain.
- Modularity: Functions help in breaking down complex tasks into smaller, more manageable parts, enhancing the modularity of your code.
- Readability: Using functions can improve the readability of your code by providing descriptive names for specific tasks or calculations.
11. Explain about default values and in return statements in functions?
Default Values in Functions:
In R, default parameter values can be assigned to function arguments, which enables you to call a function without explicitly providing a value for those parameters in certain cases. This feature is especially useful when you want to provide a commonly used value for a function argument, but still allow the flexibility for the user to override it if needed.
Syntax for Defining Default Parameter Values:
function_name <- function(arg1 = default_value1, arg2 = default_value2, ...)
{
# Function body
}
In the function declaration, you can assign default values (e.g., default_value1,
default_value2) to function arguments (e.g., arg1, `arg2), making them optional when calling the function.
Example of Default Values in a Function:
# Define a function with default parameter value
greet_user <- function(name = "Guest") {
cat(paste("Hello,", name, "Welcome to our site!"))
}
# Call the function with default value
greet_user() # Output: Hello, Guest. Welcome to our site!
# Call the function with a specific value
greet_user("Alice") # Output: Hello, Alice. Welcome to our site!
In this example:
- The
greet_user
function is declared with thename
parameter having a default value of “Guest”. - When the function is called without providing a value for
name
, it uses the default value “Guest”. - When the function is called with a specific name (e.g., “Alice”), it overrides the default value.
Return Statements in Functions:
In R, the return
statement is used to explicitly specify the value that a function should return when it is called. Although it is not always necessary to use the return
statement (the last expression evaluated before the end of a function is automatically returned), it helps in making the function’s intention clearer.
Syntax for Return Statement:
function_name <- function(arg1, arg2, ...)
{
# Function body
return(output_value) # Optional: Explicit return statement
}
The return statement can be used anywhere within the function body to return a specific value. Once a return statement is executed, the function exits and the specified value is returned.
Example of Return Statement in a Function:
# Define a function with a return statement
calculate_sum <- function(a, b) {
sum <- a + b # Calculate the sum
return(sum) # Return the calculated sum
}
# Call the function and store the returned value
result <- calculate_sum(3, 5)
cat("The sum is:", result) # Output: The sum is: 8
In this example:
- The
calculate_sum
function calculates the sum of two numbers (a
andb
) and uses the return statement to explicitly return the calculated sum. - When the function is called with arguments
3
and5
, the returned sum is stored inresult
and then printed.
12. Write about Arithmetic and Boolean operators in R programming.
Arithmetic Operators in R Programming:
Addition (+):
- Used to add two numbers or vectors.
- Example:
5 + 3
equals8
.
Subtraction (-):
- Used to subtract one number from another.
- Example:
10 - 4
equals6
.
Multiplication (*):
- Used to multiply two numbers or vectors element-wise.
- Example:
2 * 3
equals6
.
Division (/):
- Used to divide one number by another.
- Example:
12 / 3
equals4
.
Modulus (%%):
- Returns the remainder after division.
- Example:
10 %% 3
equals1
.
Boolean Operators in R Programming:
Logical AND (&&):
- Returns TRUE if both conditions are TRUE.
- Example:
TRUE && FALSE
returnsFALSE
.
Logical OR (||):
- Returns TRUE if any condition is TRUE.
- Example:
TRUE || FALSE
returnsTRUE
.
Logical NOT (!):
- Negates a logical value.
- Example:
!TRUE
returnsFALSE
.
Equality (==):
- Checks if two values are equal.
- Example:
5 == 5
returnsTRUE
.
Inequality (!=):
- Checks if two values are not equal.
- Example:
10 != 5
returnsTRUE
.
Greater Than (>):
- Checks if the left operand is greater than the right.
- Example:
8 > 3
returnsTRUE
.
Less Than (<):
- Checks if the left operand is less than the right.
- Example:
2 < 7
returnsTRUE
.
Greater Than or Equal To (>=):
- Checks if the left operand is greater than or equal to the right.
- Example:
5 >= 5
returnsTRUE
.
Less Than or Equal To (<=):
- Checks if the left operand is less than or equal to the right.
- Example:
3 <= 3
returnsTRUE
.