C# 2023 NEP Solved Question Paper (C# and .Net Framework)
Click here to Download C# .Net Framework NEP 2022 Question Paper.
Section - A
Answer any Ten questions. (10x2=20)
1. Expand CLR and CTS.
- Common Language Runtime
- Common Type System
2. Define class and objects.
Class is a collection of object that describes the properties of variable, methods and functions.
3. Write the use of write Line () and ReadLine()
The WriteLine() method takes a string as input and writes it to the console. For example, the following code will write the string “Hello, world!” to the console:
The ReadLine() method reads a line of text from the console and returns it as a string. For example, the following code will read a line of text from the console and store it in the variable input:
4. List various keywords used in C#.
abstract,as,base,break,case,catch,checked,class,const,continue,default,delegate,do,else, etc..
5. Give the syntax of try, catch block in C#.
try
{
// Code to be tried
}
catch (Exception e)
{
// Code to handle the exception
}
6. Define Events.
Events in C# are a way of notifying other objects when something happens in a specific object. Events are typically used to decouple code, making it more modular and reusable.
To define an event in C#, you use the event keyword.
7. What is Menustrip in C#?
The MenuStrip control in C# is a Windows Forms control that allows you to add a menu bar to your application. It is a replacement for the MainMenu control, which is no longer supported in .NET Core.
8. List any four relational operators in C#.
The four relational operators in C# are:
== (Equal to)
!= (Not equal to)
< (Less than)
> (Greater than)
9. Define exception in C#.
An exception in C# is an event that occurs during the execution of a program that disrupts the normal flow of the program. Exceptions can be caused by a variety of factors, such as user input errors, file not found errors, and network errors.
10. Write the syntax of Do-while loop in C#.
do
{
// Code to be executed
} while (condition);
11. Define Delegate.
A delegate in C# is a type that represents references to methods with a particular parameter list and return type. Delegates are used to pass methods as arguments to other methods.
12. Define constructors.
A constructor in C# is a special method that is called when an object of a class is created. Constructors are used to initialize the state of the object by setting the values of its fields.
Section - B
2. Answer any Three question of the following
13. Explain operators in C#.
Operators are the foundation of any programming language. Thus the functionality of C# language is incomplete without the use of operators. Operators allow us to perform different kinds of operations on operands. In C#, operators Can be categorized based upon their different functionality :
1. Arithmetic Operators
2. Relational Operators
3. Logical Operators
4. Bitwise Operators
5. Assignment Operators
6. Conditional Operator
In C#, Operators can also categorized based upon Number of Operands :
Unary Operator: Operator that takes one operand to perform the operation.
Binary Operator: Operator that takes two operands to perform the operation.
Ternary Operator: Operator that takes three operands to perform the operation.
Arithmetic Operators
These are used to perform arithmetic/mathematical operations on operands. The Binary Operators falling in this category are :
Addition: The ‘+’ operator adds two operands. For example, x+y.
Subtraction: The ‘-‘ operator subtracts two operands. For example, x-y.
Multiplication: The ‘*’ operator multiplies two operands. For example, x*y.
Division: The ‘/’ operator divides the first operand by the second. For example, x/y.
Modulus: The ‘%’ operator returns the remainder when first operand is divided by the second. For example, x%y.
// C# program to demonstrate the working
// of Binary Arithmetic Operators
using System;
namespace Arithmetic
{
class GFG
{
// Main Function
static void Main(string[] args)
{
int result;
int x = 10, y = 5;
// Addition
result = (x + y);
Console.WriteLine("Addition Operator: " + result);
// Subtraction
result = (x - y);
Console.WriteLine("Subtraction Operator: " + result);
// Multiplication
result = (x * y);
Console.WriteLine("Multiplication Operator: "+ result);
// Division
result = (x / y);
Console.WriteLine("Division Operator: " + result);
// Modulo
result = (x % y);
Console.WriteLine("Modulo Operator: " + result);
}
}
}
Output:
Addition Operator: 15
Subtraction Operator: 5
Multiplication Operator: 50
Division Operator: 2
Modulo Operator: 0
14. Explain Architecture of .NET framework.
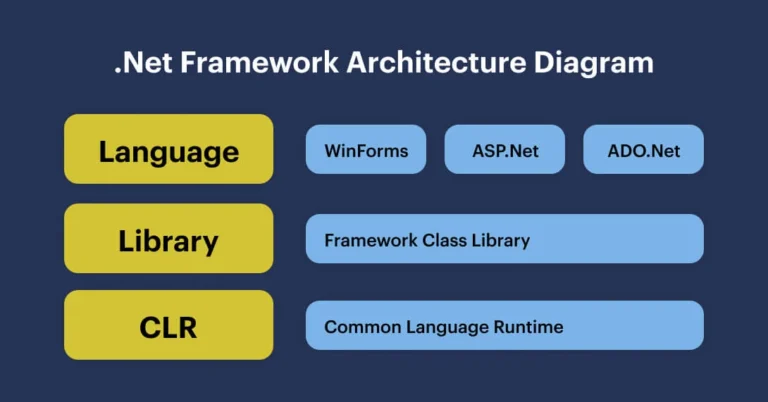
The .NET Framework is a software development framework developed by Microsoft that provides a set of libraries and tools for building and running applications on Windows. The .NET Framework architecture is based on the Common Language Runtime (CLR) and the Common Type System (CTS).
The CLR is a virtual machine that executes code written in any of the .NET-supported languages, such as C#, F#, and Visual Basic .NET.
The CLR provides a number of services to managed code, including:
- Memory management: The CLR automatically manages memory for managed code, including allocating and deallocating memory as needed.
- Security: The CLR provides a number of security features, such as code access security and sandboxing.
- Type safety: The CLR ensures that managed code is type safe, meaning that it cannot access memory in an unsafe manner.
- Performance: The CLR optimizes managed code for performance, using techniques such as just-in-time (JIT) compilation.
The CTS is a set of rules that define how types are declared, used, and managed in the CLR. The CTS provides a common set of types that can be used by all managed languages. This allows managed code written in different languages to interact with each other seamlessly.
The .NET Framework also includes a number of class libraries, which provide a set of pre-built classes and components that can be used to develop applications. The class libraries include classes for handling data storage, error handling, and other tasks that are common across all types of applications.
Here are some of the benefits of using the .NET Framework architecture:
- Language interoperability
- Performance
- Security
- Reliability
The .NET Framework is a popular choice for developing applications on Windows. By understanding the .NET Framework architecture, you can develop more efficient, secure, and reliable applications.
15. Differentiate between SDI and MDI.
SDI (Single Document Interface) | MDI (Multiple Document Interface) |
---|---|
Supports only one document at a time. | Supports multiple documents in the same window. |
Each document opens in a separate window. | Multiple documents open within a parent window. |
Simpler and lightweight interface. | More complex but allows better organization. |
Used in applications like Notepad. | Used in applications like Adobe Photoshop. |
Easier to implement and manage. | Requires additional coding for window management. |
16. Explain methods of console class in C#.
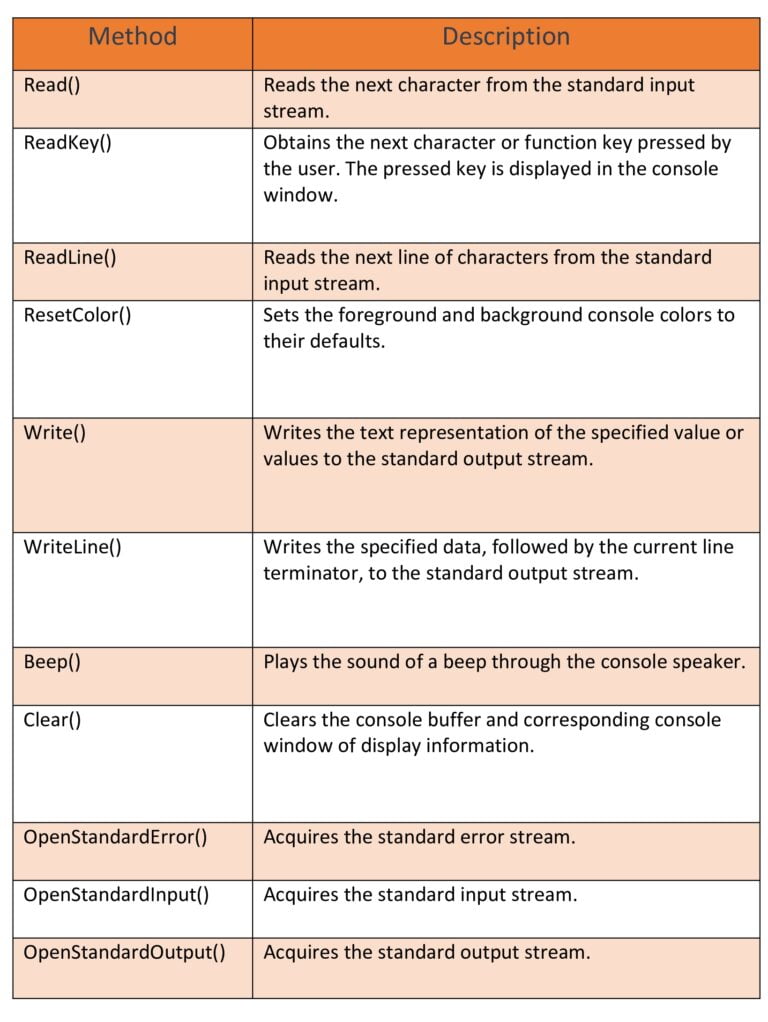
17. Write a C# program to demonstrate exception handling in C#.
using System;
class Program
{
static void Main(string[] args)
{
// Try block
try
{
// Code that may generate an exception
int x = 10;
int y = 0;
int z = x / y;
}
// Catch block
catch (DivideByZeroException ex)
{
// Handle the DivideByZeroException exception
Console.WriteLine("DivideByZeroException: {0}", ex.Message);
}
// Finally block
finally
{
// Code that is always executed, regardless of whether an exception is thrown
Console.WriteLine("Finally block executed");
}
}
}
Section - C
Answer any Two of the following. (2x10=20)
18. Explain conditional statements in C#.
1. The if Statement
Use the if statement to specify a block of C# code to be executed if a condition is True.
Syntax
if (condition)
{
// block of code to be executed if the condition is True
}
Example
int x = 20;
int y = 18;
if (x > y)
{
Console.WriteLine("x is greater than y");
}
2. The else Statement
Use the else statement to specify a block of code to be executed if the condition is False.
Syntax
if (condition)
{
// block of code to be executed if the condition is True
}
else
{
// block of code to be executed if the condition is False
}
Example
int time = 20;
if (time < 18)
{
Console.WriteLine("Good day.");
}
else
{
Console.WriteLine("Good evening.");
}
// Outputs "Good evening
3.The else if Statement
Use the else if statement to specify a new condition if the first condition is False.
Syntax
if (condition1)
{
// block of code to be executed if condition1 is True
}
else if (condition2)
{
// block of code to be executed if the condition1 is false and condition2 is True
}
else
{
// block of code to be executed if the condition1 is false and condition2 is False
}
Example
int time = 22;
if (time < 10)
{
Console.WriteLine("Good morning.");
}
else if (time < 20)
{
Console.WriteLine("Good day.");
}
else
{
Console.WriteLine("Good evening.");
}
// Outputs "Good evening."
4.C# Switch Statements
Use the switch statement to select one of many code blocks to be executed.
Syntax
switch(expression)
{
case x:
// code block
break;
case y:
// code block
break;
default:
// code block
break;
}
5.C# For Loop
When you know exactly how many times you want to loop through a block of code, use the for loop instead of a while loop:
Syntax
for (statement 1; statement 2; statement 3)
{
// code block to be executed
}
Statement 1 is executed (one time) before the execution of the code block.
Statement 2 defines the condition for executing the code block.
Statement 3 is executed (every time) after the code block has been executed.
The example below will print the numbers 0 to 4:
Example
for (int i = 0; i < 5; i++)
{
Console.WriteLine(i);
}
6.C# Break and Continue
C# Break
You have already seen the break statement used in an earlier chapter of this tutorial. It was used to "jump out" of a switch statement.
The break statement can also be used to jump out of a loop.
This example jumps out of the loop when i is equal to 4:
Example
for (int i = 0; i < 10; i++)
{
if (i == 4)
{
break;
}
Console.WriteLine(i);
}
7.C# Continue
The continue statement breaks one iteration (in the loop), if a specified condition occurs, and continues with the next iteration in the loop.
This example skips the value of 4:
Example
for (int i = 0; i < 10; i++)
{
if (i == 4)
{
continue;
}
Console.WriteLine(i);
}
19. Explain C# methods with example.
A method is a block of code which only runs when it is called.
You can pass data, known as parameters, into a method.
Create a Method
class Program
{
static void MyMethod()
{
// code to be executed
}
}
Example Explained
MyMethod() is the name of the method
static means that the method belongs to the Program class and not an object of the Program class. You will learn more about objects and how to access methods through objects later in this tutorial.
void means that this method does not have a return value. You will learn more about return values later in this chapter
Call a Method
To call (execute) a method, write the method’s name followed by two parentheses () and a semicolon;
In the following example, MyMethod() is used to print a text (the action), when it is called:
Example
Inside Main(), call the myMethod() method:
static void MyMethod()
{
Console.WriteLine("I just got executed!");
}
static void Main(string[] args)
{
MyMethod();
}
// Outputs “I just got executed!”
20. Explain
a.Boxing and unboxing
Boxing
The process of converting a Value Type variable (char, int etc.) to a Reference Type variable (object) is called Boxing.
Boxing is an implicit conversion process in which object type (super type) is used.
Value Type variables are always stored in Stack memory, while Reference Type variables are stored in Heap memory.
Example :
int num = 23; // 23 will assigned to num
Object Obj = num; // Boxing
Unboxing
The process of converting a Reference Type variable into a Value Type variable is known as Unboxing.
It is an explicit conversion process.
Example :
int num = 23; // value type is int and assigned value 23
Object Obj = num; // Boxing
int i = (int)Obj; // Unboxing
// C# implementation to demonstrate
// the Boxing and Unboxing
using System;
class GFG
{
// Main Method
static public void Main()
{
// assigned int value
// 23 to num
int num = 23;
// boxing
object obj = num;
// unboxing
int i = (int)obj;
// Display result
Console.WriteLine("Value of ob object is : " + obj);
Console.WriteLine("Value of i is : " + i);
}
}
b. Dictionary in C#.
a Dictionary is a collection type that represents a collection of key-value pairs. Each key in a Dictionary must be unique, and it is used to access its corresponding value. Dictionaries are commonly used to store and retrieve data efficiently based on a specific key.
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// Creating a Dictionary with string keys and int values
Dictionary<string, int> ageDictionary = new Dictionary<string, int>();
// Adding key-value pairs to the Dictionary
ageDictionary["Alice"] = 28;
ageDictionary["Bob"] = 35;
ageDictionary["Charlie"] = 42;
ageDictionary["David"] = 22;
// Accessing values by keys
Console.WriteLine("Alice's age is " + ageDictionary["Alice"]);
Console.WriteLine("Bob's age is " + ageDictionary["Bob"]);
// Checking if a key exists in the Dictionary
string keyToCheck = "Eve";
if (ageDictionary.ContainsKey(keyToCheck))
{
Console.WriteLine(keyToCheck + "'s age is " + ageDictionary[keyToCheck]);
}
else
{
Console.WriteLine(keyToCheck + " is not found in the Dictionary.");
}
// Iterating through the Dictionary
Console.WriteLine("Dictionary contents:");
foreach (var pair in ageDictionary)
{
Console.WriteLine(pair.Key + " is " + pair.Value + " years old.");
}
}
• Dictionary<string, int> dictionary = new Dictionary<string, int>();
• You can add key-value pairs to a dictionary using the Add() method. The Add() method takes two parameters: the key and the value.
The following example shows how to add key-value pairs to the dictionary:
dictionary.Add("Alice", 1);
dictionary.Add("Bob", 2);
dictionary.Add("Charlie", 3);
Supper explain … I have 5 star in this question peper
the 15th answer mdi and sdi difference is completely unrelated to the topic
SDI and MDI difference is totally different from topic